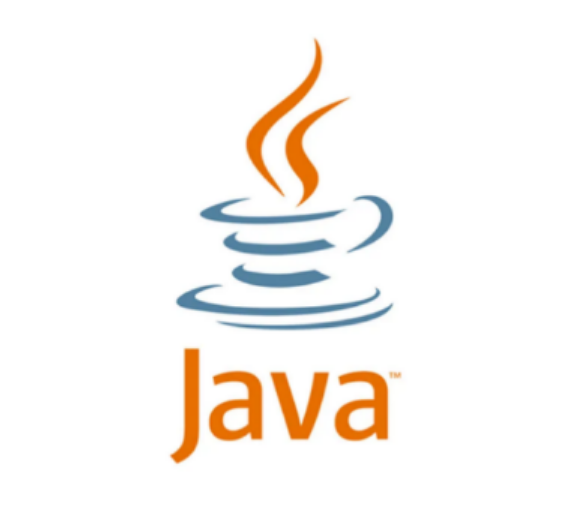
728x90
- Object클래스는 모든 클래스의 최상위 클래스
- 아무것도 상속받지 않으면 자동으로 Object를 상속
- Object가 가지고 있는 메소드는 모든 클래스에서 다 사용할 수 있다는 것을 의미
Object클래스에서 가장 많이 사용되는 메소드는 다음과 같다.
- equals : 객체가 가진 값을 비교할 때 사용
- toString : 객체가 가진 값을 문자열로 반환
- hashCode : 객체의 해시코드 값 반환
그런데 이 세가지 메소드는 사용할 때 반드시 오버라이딩해서 사용해야 한다.
예를 들어 보자
같은 클래스를 통해 만들어준 s1과 s2라는 인스턴스에 모두 같은 값을 집어넣고 equals를 이용해 비교하면 어떻게 될까?
import java.util.Objects;
public class Student {
String name;
String number;
int birthYear;
public static void main(String[] args) {
// s1과 s2에 모두 동일한 값을 집어 넣는다.
Student s1 = new Student();
s1.name = "홍길동";
s1.number = "1234";
s1.birthYear = 1995;
Student s2 = new Student();
s2.name = "홍길동";
s2.number = "1234";
s2.birthYear = 1995;
if(s1.equals(s2))
System.out.println("s1 == s2");
else
System.out.println("s1 != s2");
}
}
답은 "s1 != s2"가 출력되는 것을 볼 수 있다.
s1과 s2의 해시코드를 비교해도 서로 다른 값을 가지고 있는 것을 볼 수 있다.
이것은 현재 equals메소드와 hashCode메소드는 Object가 구현하고 있는 메소드를 그냥 사용하고 있기 때문이다.
equals메소드와 hashCode메소드는 개발자가 알맞게 오버라이딩하여 사용하여야 한다.
인텔리제이나 이클립스 같은 IDE를 사용하면 손쉽게 오버라이딩을 할 수 있다.
import java.util.Objects;
public class Student {
String name;
String number;
int birthYear;
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
Student student = (Student) o;
return Objects.equals(number, student.number);
}
@Override
public int hashCode() {
return Objects.hash(number); //해시코드를 구하는 코드
}
@Override
public String toString() {
return "Student{" +
"name='" + name + '\'' +
", number='" + number + '\'' +
", birthYear=" + birthYear +
'}';
}
public static void main(String[] args) {
Student s1 = new Student();
s1.name = "홍길동";
s1.number = "1234";
s1.birthYear = 1995;
Student s2 = new Student();
s2.name = "홍길동";
s2.number = "1234";
s2.birthYear = 1995;
if(s1.equals(s2))
System.out.println("s1 == s2");
else
System.out.println("s1 != s2");
}
}
이렇게 오버라이딩을 한 후 비교해 보니 아까와는 다르게
"s1 == s2"
가 출력된 것을 확인할 수 있었다.
s1과 s2의 해시코드를 비교해도 서로 같은 값을 가지고 있는 것을 볼 수 있다.
'JAVA' 카테고리의 다른 글
Generic(제네릭) (0) | 2021.07.15 |
---|---|
StringBuffer(스트링버퍼) (0) | 2021.07.13 |
Finally와 Try with Resource (0) | 2021.07.11 |
인터페이스(INTERFACE)보충 및 다형성(POLYMORHPISM) (0) | 2021.07.11 |
스태틱(STATIC) (0) | 2021.07.05 |